React Native SDK
The React Client SDK helps user submit their bank statements via upload or net banking credentials in your React application.
#
See BankConnect in actionThe demo video below shows how a user submit bank statement using net banking credentials:
The video below shows a user submit bank statement by uploading the PDF file:
#
Integration WorkflowThe diagram below illustrates the integration workflow in a nutshell:
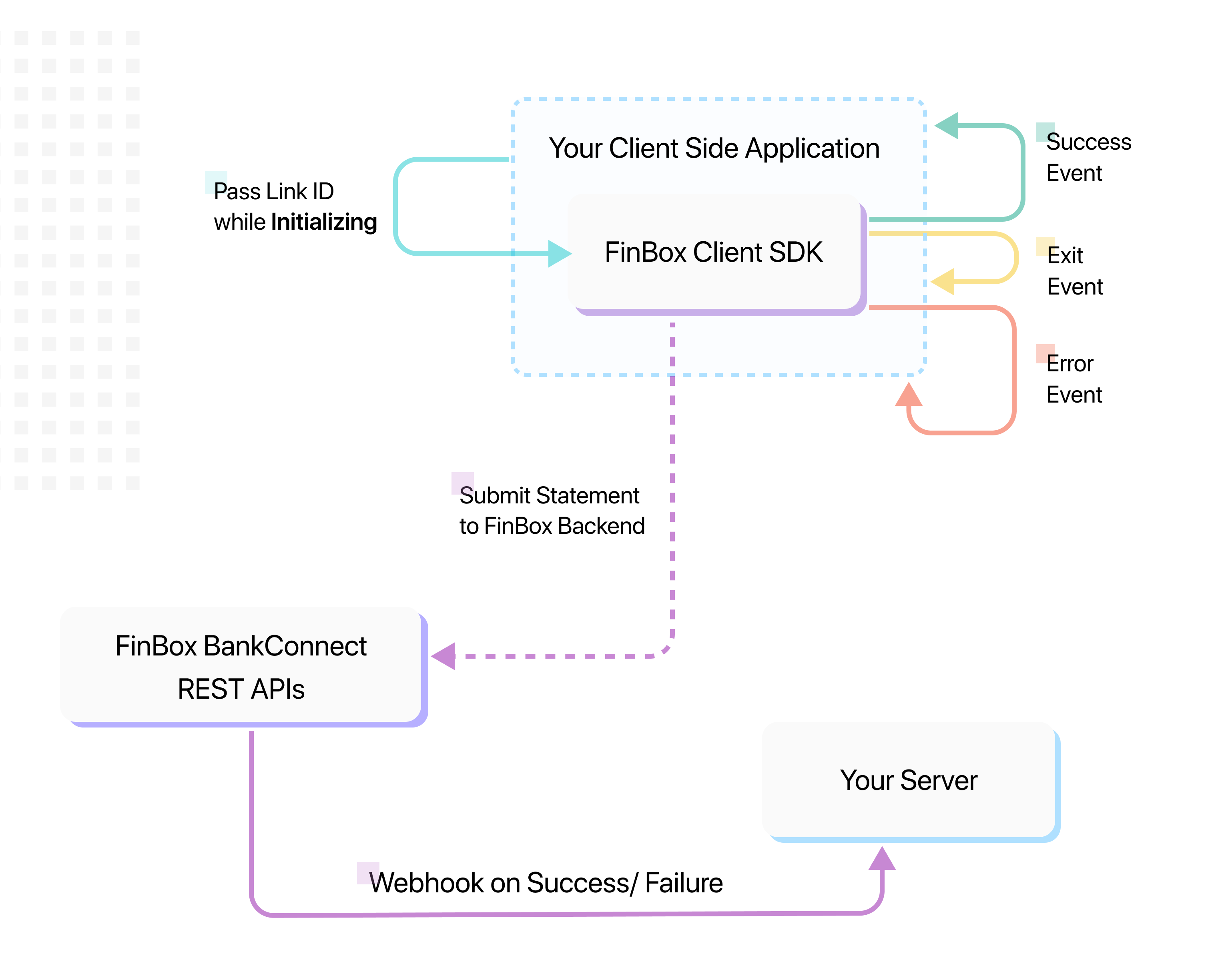
#
Sample ProjectWe have hosted a sample project on GitHub, you can check it out here:
#
Including the libraryThe library is available as an npm package. To install the dependency
After installing this, you have to install an additional dependency, you can skip this if its already part of your project:
Once the dependency is added. You can use a BankConnect view anywhere in your component. Since the SDK opens a modal it is recommended to add it to the top hierarchy of you View.
Attribute | Description | Required |
---|---|---|
linkId | unique identifier for the user. The view will not work if linkId is not specified | Yes |
apiKey | holds the unique API Key provided. | Yes |
onExit | specifies the callback when the user exits the SDK | No, but recommended |
onSuccess | specifies the callback when the document has been successfully uploaded. | No, but recommended |
onError | specifies the callback when an error occurred | No, but recommended |
fromDate and toDate | specifies the time period for which the statements will be fetched. If not provided the default date range is 6 months from the current date. Its format should be in dd/MM/yyyy . | No |
bankName | pass the bank identifier to skip the bank selection screen and directly open a that bank's screen instead | No |
fromDate
and toDate
specify the period for which the statements will be fetched. For example, if you need the last 6 months of statements, fromDate
will be today's date - 6 months and toDate
will be today's date - 1 day. If not provided the default date range is 6 months from the current date. It should be in dd/MM/yyyy
format.
Period Values
- Please make sure
fromDate
is always less thantoDate
- Make sure
toDate
is never today's date, the maximum possible value for it is today's date - 1 day
#
CallbackThis section lists the different callbacks:
#
SuccessonSuccess
function will be called when the document has been successfully uploaded.
payload
object will have following structure:
Key | Description |
---|---|
entityId | Unique identifier used for fetching statement data |
linkId | Identifier passed while initializing the SDK |
#
ExitOnExit
function will be called when the user exits the SDK.
payload
object will have following structure:
Key | Description |
---|---|
linkId | Identifier passed while initializing the SDK |
#
ErrorOnError
function will be called whenever any error occurs in the user flow.
payload
object will have following structure:
Key | Description |
---|---|
linkId | Identifier passed while initializing the SDK |
reason | Reason for failure |
Webhook
To track additional errors, and transaction process completion at the server-side, it is recommended to also integrate Webhook.
#
Error typesIn case of Error, error_type of MUXXX
implies an error in Manual PDF Upload and NBXXX
implies its from Netbanking.
Case | error_type | Sample payload |
---|---|---|
Trial Expired for Dev Credentials | MU002 | {"reason:"Trial Expired for Dev Credentials",linkID:"<USER_ID_PASSED>","error_type":"MU002"} |
PDF Password Incorrect | MU003 | {"reason:"Password Incorrect",linkID:"<USER_ID_PASSED>","error_type":"MU003"} |
Specified bank doesn't match with detected bank | MU004 | {"reason:"Not axis statement",linkID:"<USER_ID_PASSED>","error_type":"MU004"} |
Non Parsable PDF - PDF file is corrupted or has no selectable text (only scanned images) | MU006 | {"reason:"",linkID:"<USER_ID_PASSED>","error_type":"MU006"} |
Not a valid statement or bank is not supported | MU020 | {"reason:"Not a valid statement or bank is not supported",linkID:"<USER_ID_PASSED>","error_type":"MU020"} |
Invalid Date Range | MU021 | {"reason:"Please upload statements within the date range fromDate to toDate",linkID:"<USER_ID_PASSED>","error_type":"MU021"} |
NetBanking Failed | NB000 | {"reason:"failure_message",linkID:"<USER_ID_PASSED>","error_type":"NB000"} |
Netbanking Login Error | NB003 | {"reason:"failure_message",linkID:"<USER_ID_PASSED>","error_type":"NB003"} |
Captcha Error | NB004 | {"reason:"Invalid Captcha",linkID:"<USER_ID_PASSED>","error_type":"NB004"} |
Security Error | NB005 | {"reason:"failure_message",linkID:"<USER_ID_PASSED>","error_type":"NB005"} |
#
EventsAndroid and JS events are passed which can used for purposes such as analytics.The object passed is of the following format.
Event | status | data |
---|---|---|
Bank selected | bank_selected | <BANK NAME> |
Manual upload screen opened | manual | - |
Clicked back in Manual Upload | manual_upload_back | - |
Clicked back in Netbanking | net_banking_back | - |
Above Events can be recieved in two ways
- Listen to window postMessage via
target.addEventListener("message", (event) =>{});
- In case the SDK is used in a Android WebView. A Javascript Interface can be used to get the events.
- Interface Name:
BankConnectAndroid
- Callback Function:
onResult
.